Docker has been a game changer in how software is developed, shared, and deployed. It allows developers to package their applications into containers for ease of shipping and deployment. To embrace this new way of application deployment, it has become necessary to learn how to work with docker. In this article, we will explore how to get started with docker by highlighting the common docker commands, Dockerfile format, and docker playgrounds that you can use to practice running docker commands.
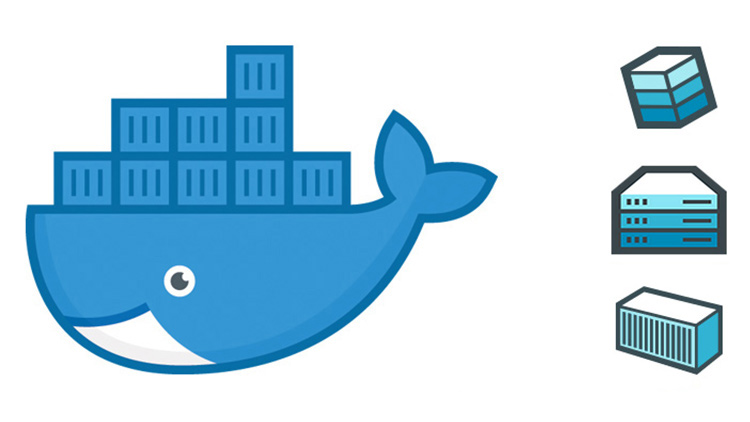
Getting started with docker
In a previous guide, we covered how to install docker on Linux: See the article here: How to Install docker on Linux. Refer to the official documentation for more Docker documentation
What is a Dockerfile?
For an image to come into being, a Dockerfile is used. A Dockerfile contains a set of instructions that defines the steps and configurations required to create a portable containerized environment. The common directives are:
- Copy files
- Install dependencies
- Expose network ports
- Declare the base Image
- Declare the working directory
- Declare the command that runs the application
Given below is an example of a Dockerfile that can be used to Dockerize a Django application. Note that for a Dockerfile to be valid, the name should strictly be Dockerfile
FROM python:3
ENV PYTHONUNBUFFERED 1
WORKDIR /app
COPY requirements.txt ./
RUN pip install --upgrade pip
RUN pip install -r requirements.txt
COPY . ./
EXPOSE 8000
CMD ["python", "manage.py", "runserver", "0.0.0.0:8000"]
Can I practice docker online?
The answer is yes! You may not need to install docker on your machine locally. There exist hundreds of online docker playgrounds that you can choose from, some are free while others are premium. To get a free Docker lab online, visit Free Docker playground, log in, and launch a Docker lab.
Mastering Essential Docker Commands
In this section, we have highlighted the common docker commands. Explore them.
Run a docker image
docker run <image-name> #pulls and image and starts a new container from a Docker image.
example: docker run nginx #pulls nginx image from docker hub and starts it
Get a list of running containers
docker ps #Lists all running containers.
List images in docker
docker images #Lists all available Docker images on your system.
Stop a container in docker
docker stop <container_id> #Stops a running container.
example: docker stop 6bea #stops container whose ID starts with 6bea. The container name can also be used in place of the container ID
Start a container in docker
docker start <container_id> #Starts a stopped container.
example: docker start 6bea #starts the container whose ID starts with 6bea.
Restart a container
docker restart <container_id> #Stops and starts a container.
example: docker restart 6bea #stops and restarts container with ID 6bea
Remove or delete a container
docker rm <container_id> #Removes a stopped container. If the container is still running, either stop it or apply the force flag
example: docker rm 6bea #removes a docker container with id 6bea; if not running.
docker ps -aq #This command lists all container IDs, one per line.
docker rm -f #Removes the specified containers. The -f flag is used to force the removal of running containers.
docker rm -f $(docker ps -aq) #forcefully remove all containers
Remove or delete an image
docker rmi <docker_image> #Removes a Docker image.
example: docker rmi nginx #removes an image named nginx
docker rmi $(docker images -a -q) #removes all images. To force removal of images in use, use below code:
docker rmi $(docker images -a -q) --force
docker image prune -a # removes all images apart from those in use
Execute commands on a docker container
docker exec <container_id> <command> #This executes a command in a running container.
example: docker exec 6bea cat /home/info.txt #This command executes the cat command and displays the content of the file name info.txt under the home directory.
Build an image
docker build -t <image_name> . #Builds a Docker image from a Dockerfile.
example: docker build -t mypointofsale . #this builds a docker image from a Dockerfile and names it mypointofsale. Note the dot(.) which indicates that the docker files and project files are in the current directory.
View container logs
docker logs <container_id> #Displays the logs of a running container.
example: docker logs 6bea #displays the logs of container with ID 6bea
Inspect a container
docker inspect <container_id> #Returns low-level information about a container or image.
example: docker inspect 6bea #Retuns low-level information about a container with id 6bea
Pull an image
docker pull <image_name> #Downloads a Docker image from a registry but does not start it.
example: docker pull nginx #downloads nginx image from the docker registry.
Publish an image
docker push <image_name> #Pushes a Docker image to a registry. Usually, this is an image on your local machine. Ensure the image name starts with your docker username.
example: docker push <docker_username/mypointofsale> #pushes the image mypointofsale to docker regity with the username docker_username.
Tag docker images
docker tag <current_image_name> <new_image_name>: Tags a Docker image with a new repository name and tag.
example: docker tag mypointofsale:latest docker_username/mypointofsale:latest #creates a new copy of mypointofsale image and names it docker_username/mypointofsale with the tag latest
How to get real-time resource usage
docker stats <container_id> #Displays real-time resource usage statistics of containers.
example: docker stats 6bea #displays real-time resource usage statistics of a container with id 6bea
Run docker commands on docker container terminal
docker exec -it <container_id> /bin/bash #access the docker container on commandline. Here, you can execute linux commands.
example: docker exec -it 6bea /bin/bash #access the docker container on commandline for container with ID 6bea
Copy content from docker host to a docker container
docker cp <local_path_to_file> <container_id_or_name>:<container_path>
example: docker cp /tmp/data.xml 6bea:/usr/data #copies a file <data.xml> from the tmp folder to docker container with ID 6bea under the path /usr/data
Copy content from a docker container to a docker host
docker cp <container_id_or_name>:/path/to/container/directory /path/to/host/directory
Give a user permission to run docker.
Case: Allow a newly created user to run docker commands without using sudo
sudo groupadd docker #add a docker group if it does not exist
sudo usermod -aG docker new-user #add new user to the docker group
##To test if new-user can run docker commands; switch to the new user and run
su new-user #switch to new-user
docker --version #check docker version with new user. Run any other command with the new user to confirm.
Create an Image from a Container
Use the docker commit
command to create an image from the running container. Replace container_id_or_name
with the actual ID or name of the container you want to create an image from, and use the desired image name and tag:
docker commit container_id_or_name image-name:tag
Create the Docker Network
docker network create --driver=DRIVER-TYPE --subnet=IP-SUBNET --ip-range=IP-RANGE network-name
common docker network drivers
- Bridge: The default network driver. It creates an internal private network on the host machine and provides communication between containers on the same host.
- Host: This network mode removes network isolation between the Docker container and the Docker host. Containers share the host’s networking namespace, meaning they can directly access services running on the host and vice versa.
- None: In this mode, the container is not attached to any network. You have to manually configure the network for the container, or use other mechanisms like Docker exec to access the container.
Save a docker image as an archive
docker save -o archive-name.tar image-name:tag #This saves an image as an archive.
Load a docker image from an archive
docker load -i archive-name.tar #This loads an image from the archive. It retains the same name and tag from the original image.
Running a container in the background
When you run Docker containers with Ubuntu or other operating systems, the default behavior is that the container will start and run the primary command specified in the Docker image (usually CMD or ENTRYPOINT in the Dockerfile). Once that command finishes or exits, the container will stop. To prevent the container from exiting, you can run it in the background (detached mode) and ensure there’s a process that keeps running, such as a shell or a long-running service. Example:
docker run -d ubuntu tail -f /dev/null
docker exec -it ubuntu-mysql bash
In conclusion, Docker commands are the building blocks of Docker’s functionality, allowing you to manage containers, images, and other aspects of your containerized applications. With familiarity with docker commands, you gain the ability to streamline your development workflow, and application deployment, and troubleshoot containerized environments effectively. In case of a concern, do a comment in the comment section.
Web Hosting and email hosting Packages
Related content
- A Practical Tutorial for Dockerizing Software Applications
- How to Configure a Docker App to a Domain Name
- Getting Started with Docker | Docker commands
- How To Run Scripts in Linux
- Deploy a Django Application on EC2 Instance with Nginx
- How to configure a domain to a docker container and install an SSL certificate on AWS